Yes, the Interpreter is a behavioral design pattern that falls under the category of the behavioral design pattern in software development. The Interpreter pattern provides a way to evaluate and interpret grammar or expressions of a language. It allows us to define a language, parse it, and execute the instructions. This pattern is useful when we need to create a custom language or interpret some kind of language-like syntax.
The Interpreter pattern involves two main components: the context and the expression. The context is the object that contains the information that needs to be interpreted. The expression represents a single unit of the language or grammar, and it provides a way to interpret the context.
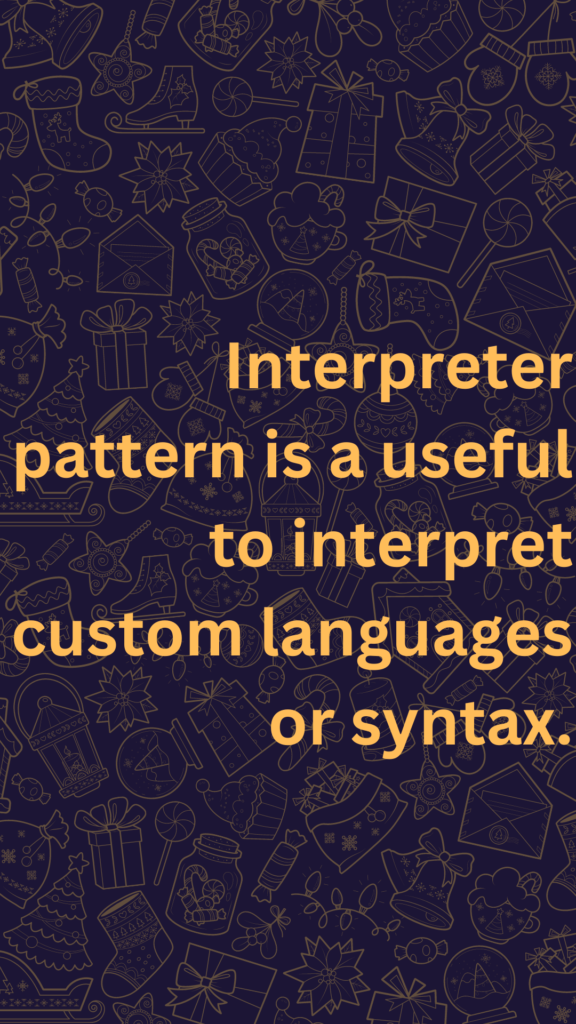
The Interpreter pattern can be implemented in several ways. One of the most common ways is to use an Abstract Syntax Tree (AST) to represent the grammar or language. The AST represents the structure of the language in a hierarchical way. Each node in the tree represents an expression or a statement, and it has its own interpreter to evaluate it.
To implement the Interpreter pattern, we need to define the grammar or language that we want to interpret. We need to define the syntax of the language, the rules for parsing it, and the actions that need to be performed for each expression or statement. Once we have defined the grammar, we can create the AST and the corresponding interpreters.
The Interpreter pattern has several advantages. First, it allows us to create custom languages or syntax that can be used to express complex expressions or algorithms. Second, it separates the interpretation logic from the rest of the code, making it easier to maintain and modify. Third, it can be used to create DSLs (Domain-Specific Languages) that are tailored to specific domains, making it easier to communicate with domain experts and stakeholders.
The Interpreter pattern can be implemented in several ways. One of the most common ways is to use an Abstract Syntax Tree (AST) to represent the grammar or language. The AST represents the structure of the language in a hierarchical way. Each node in the tree represents an expression or a statement, and it has its own interpreter to evaluate it.
To implement the Interpreter pattern, we need to define the grammar or language that we want to interpret. We need to define the syntax of the language, the rules for parsing it, and the actions that need to be performed for each expression or statement. Once we have defined the grammar, we can create the AST and the corresponding interpreters.
The Interpreter pattern has several advantages. First, it allows us to create custom languages or syntax that can be used to express complex expressions or algorithms. Second, it separates the interpretation logic from the rest of the code, making it easier to maintain and modify. Third, it can be used to create DSLs (Domain-Specific Languages) that are tailored to specific domains, making it easier to communicate with domain experts and stakeholders.
What is Abstract Syntax Tree in detail?
An Abstract Syntax Tree (AST) is a data structure used by compilers, interpreters, and other tools that operate on source code. The AST is a hierarchical representation of the structure of the source code, which is used to facilitate analysis and manipulation of the code.
In this article, we will explore what an AST is, how it is constructed, and how it is used.
What is an AST?
An AST is a tree-like data structure that represents the structure of a program in a structured and hierarchical way. The AST is constructed by parsing the source code of the program, and then converting the parsed code into a tree structure.
Each node in the AST represents a construct in the source code, such as a function, a variable declaration, an expression, or a control flow statement. The nodes are connected by edges, which represent the relationships between the constructs in the code.
The AST can be thought of as a kind of “abstracted” version of the source code, which is easier to analyze and manipulate than the raw source code itself.
How is an AST constructed?
The process of constructing an AST typically involves several stages. First, the source code is parsed using a parser, which converts the raw source code into a structured representation called a parse tree.
The parse tree is then transformed into an AST by removing redundant information and reorganizing the nodes to better reflect the structure of the program. This process is called “abstracting” the syntax of the code.
During this process, the AST is usually augmented with additional information, such as type information, which is inferred from the source code. This information is used to help analyze and manipulate the code more effectively.
The resulting AST is a compact and structured representation of the program that can be used for a variety of purposes, such as optimization, analysis, and code generation.
How is an AST used?
ASTs are used by a wide variety of tools that operate on source code, including compilers, interpreters, static analyzers, and code editors.
One of the primary uses of an AST is in the optimization and analysis of code. By analyzing the structure of the code, it is possible to identify potential optimizations and generate more efficient code.
For example, an AST-based optimizer might identify code that can be replaced with a more efficient equivalent, or identify code that can be executed in parallel.
ASTs are also used in the implementation of programming languages and other tools that operate on source code. For example, an interpreter might use an AST to evaluate the code, or a compiler might use an AST to generate machine code.
Finally, ASTs are also used by code editors and other development tools to provide advanced features such as code completion, error highlighting, and refactoring.
Conclusion
In summary, an Abstract Syntax Tree (AST) is a hierarchical representation of the structure of source code. The AST is constructed by parsing the source code and converting it into a structured tree-like data structure.
ASTs are used by a wide variety of tools that operate on source code, including compilers, interpreters, static analyzers, and code editors. They are a powerful tool for analyzing and manipulating source code, and are an essential component of modern programming languages and development tools.